Composer
Composer is a tool for dependency management in PHP. It allows you to declare the libraries your project depends on and it will manage (install/update) them for you.
source getcomposer.org
Official download getcomposer.orgPackage list for composer : packagist.org
- It require PHP installation first.
- It require extension openssl enabled in php.ini (extension=openssl).
- It require extension path configurated in php.ini (extension_dir = "ext").
Basic composer workflow
download xml source
Composer will work with 2 files:
Composer use a config file compose.json to store all informations linked to project packages. It can be manually edited, but most of the time it is auto filled with composer command to add/update/remove packages.
Composer use a cache file compose.lock to prevent useless verificaction when composer install command is used.
Those files are in the root folder of the project.
Installed package will be in the folder /vendor/* of the project.
Most of the time you wont have to go in this folder, all packages are autonomous.
The good pratice is to exclude this folder from versionning server to prevent useless storage.
Semantic Versioning
Composer depencies are using the Semantic Versioning.Semantic Versioning official link : semver.org
Given a version number MAJOR.MINOR.PATCH, increment the:
MAJOR version when you make incompatible API changes,
MINOR version when you add functionality in a backwards-compatible manner, and
PATCH version when you make backwards-compatible bug fixes.
Additional labels for pre-release and build metadata are available as extensions to the MAJOR.MINOR.PATCH format.
Semantic Versioning Selectors
Symbol | Description |
---|---|
> | Upper than |
>= | Upper or equal to |
* | joker for all |
~ | any patch version from minor version |
^ | any minor version from major version |
|| | or |
Semantic Versioning Selectors examples
Symbol | Description |
---|---|
>3.3.5 | Upper than version 3.3.5 |
>=3.3.5 | Upper or equal to 3.3.5 |
3.3.* | Any patch of 3.3 version |
~3.3.5 | Any patch upper than 3.3.5 version |
^3.3.5 | From 3.3.5 to 4.0 (excluded)|
^1.0||^2.0 | From 1.0 to 3.0 (exluded) |
Semantic Versioning checker
Packagist Semver Checker semver.mwl.beManual installation
Manual installation on Windows
The following command is from the official composer website, it will install composer where you launch the command. You can se it in a .bat to automatize the process.copy to clipboard
php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');" php -r "if (hash_file('SHA384', 'composer-setup.php') === '544e09ee996cdf60ece3804abc52599c22b1f40f4323403c44d44fdfdd586475ca9813a858088ffbc1f233e9b180f061') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;" php composer-setup.php php -r "unlink('composer-setup.php');"
example of .bat to install in C:\dev\composer copy to clipboard
cd C:\dev mkdir composer cd composer php -r "copy('https://getcomposer.org/installer', 'composer-setup.php');" php -r "if (hash_file('SHA384', 'composer-setup.php') === '544e09ee996cdf60ece3804abc52599c22b1f40f4323403c44d44fdfdd586475ca9813a858088ffbc1f233e9b180f061') { echo 'Installer verified'; } else { echo 'Installer corrupt'; unlink('composer-setup.php'); } echo PHP_EOL;" php composer-setup.php php -r "unlink('composer-setup.php');"
Composer shortcut in dos
if you want to call composer in dos you have to do the command php C:\dev\composer\composer.pharA shortcut can be made creating a composer.bat with the command in it : copy to clipboard
@php "%~dp0composer.phar" %*put this in the same folder C:\dev\composer\and this folder require to be in windows PATH (composer.bat and composer.phar should be in same folder and in the PATH for some tools user composer)
now you can call composer using the command: copy to clipboard
composer
- %* equal all parameters in command line .bat files.
- You can add composer to the Path with the dos command set PATH=%PATH%;C:\dev\composer;
Manual installation on Linux
Download composer.phar and move it to local bin :copy to clipboard
curl -sS https://getcomposer.org/installer | php mv composer.phar /usr/local/bin/composer.pharCreate an alias into ~/.bashrc
copy to clipboard
alias composer='/usr/local/bin/composer.phar'Update bashrc to include the new alias
copy to clipboard
. ~/.bashrcYou can now test if it work in bash
copy to clipboard
composer
- .bashrc (in home) is the bash configuration file, each user as is own .bashrc file
Composer main commands
If composer is configurated as said before, it can be launched from anywhere using the command composer on both Windows or Linux.
So the next part will be applicable on both operating system.
To display composer command list you can just run the composer command :
copy to clipboard
copy to clipboard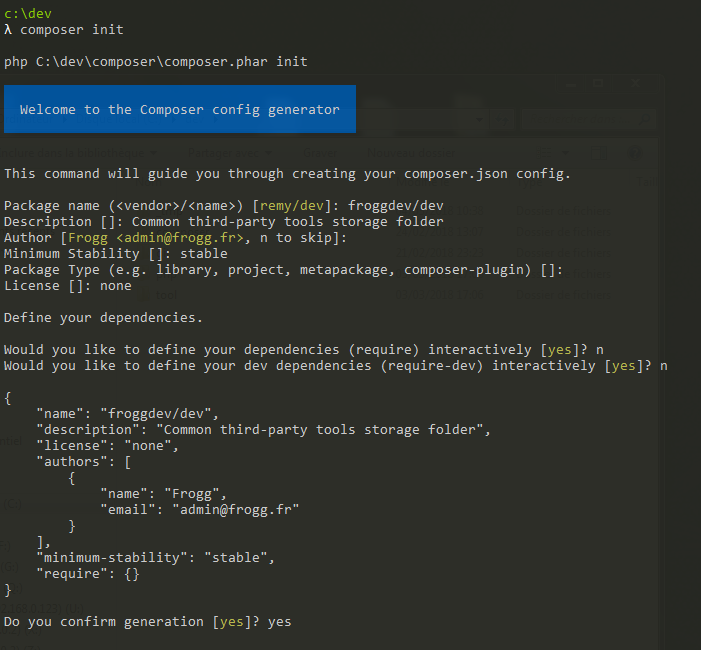
Once done a composer.json will be create and use for install PHP libraries.
There are several applications for this:
package can be installed only in dev package with the option --dev
copy to clipboard
example with dev only component : copy to clipboard
If there is a composer.lock file in the current directory, it will use the exact versions from there instead of resolving them. This ensures that everyone using the library will get the same versions of the dependencies.
If there is no composer.lock file, Composer will create one after dependency resolution.
This will resolve all dependencies of the project and write the exact versions into composer.lock.
The update command can be risky if new updated dependencies are not compatible with the current project.
To prevent update trouble, unit test and fonctionnal test has to be done after an update before validating the news changes in dependencies.
The color coding is as such:
After removing the requirements, the modified requirements will be uninstalled.
All versions of each package are checked for stability, and those that are less stable than the minimum-stability setting will be ignored when resolving your project dependencies. (Note that you can also specify stability requirements on a per-package basis using stability flags in the version constraints that you specify in a require block (see package links for more details).
Both install or update support the --no-dev option that prevents dev dependencies from being installed.
for example the key optimize-autoloader enable autoloader caching file for production environement.
So the next part will be applicable on both operating system.
To display composer command list you can just run the composer command :
copy to clipboard
composerComposer use a configuration file called composer.json, you can find the full schema on official website : composer.json schema
Initializing composer folder (optional)
composer can be initialized in a folder by the command :copy to clipboard
composer init
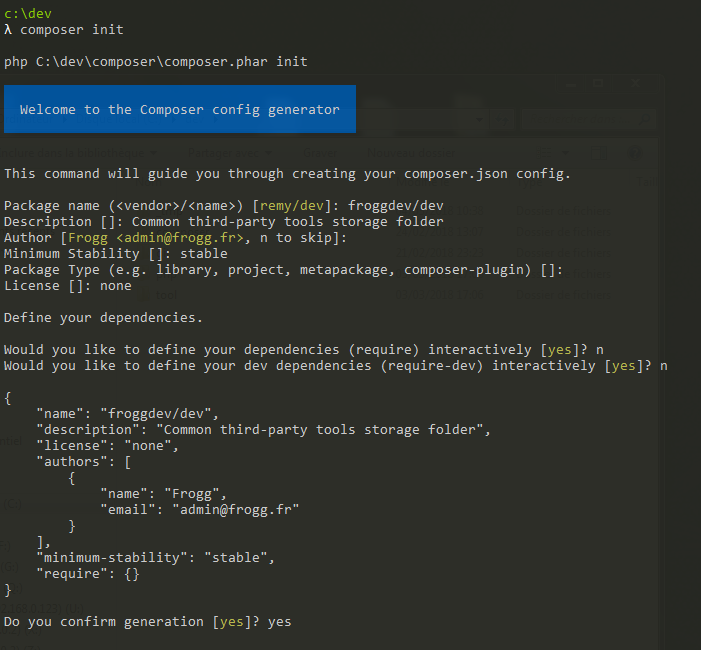
Once done a composer.json will be create and use for install PHP libraries.
create-project
You can use Composer to create new projects from an existing package. This is the equivalent of doing a git clone/svn checkout followed by a composer install of the vendors.There are several applications for this:
- You can deploy application packages.
- You can check out any package and start developing on patches for example.
- Projects with multiple developers can use this feature to bootstrap the initial application for development.
- To create a new project using Composer you can use the create-project command. Pass it a package name, and the directory to create the project in. You can also provide a version as third argument, otherwise the latest version is used.
composer create-project symfony/website-skeleton {PROJECTNAME}
require
The require command adds new packages to the composer.json file from the current directory. If no file exists one will be created on the fly.package can be installed only in dev package with the option --dev
copy to clipboard
composer require symfony/workflow
example with dev only component : copy to clipboard
composer require server --dev
install/update
install
copy to clipboardcomposer installThe install command reads the composer.json file from the current directory, resolves the dependencies, and installs them into vendor.
If there is a composer.lock file in the current directory, it will use the exact versions from there instead of resolving them. This ensures that everyone using the library will get the same versions of the dependencies.
If there is no composer.lock file, Composer will create one after dependency resolution.
update
copy to clipboardcomposer updateIn order to get the latest versions of the dependencies and to update the composer.lock file, you should use the update command. This command is also aliased as upgrade as it does the same as upgrade does if you are thinking of apt-get or similar package managers.
This will resolve all dependencies of the project and write the exact versions into composer.lock.
Important
When a project is new without composer.lock the install command will launch the update command, and create the composer.lock. This file will contain all dependency references used in the project so it need to be commited on versionning server too.The update command can be risky if new updated dependencies are not compatible with the current project.
To prevent update trouble, unit test and fonctionnal test has to be done after an update before validating the news changes in dependencies.
outdated
copy to clipboardcomposer outdatedThe outdated command shows a list of installed packages that have updates available, including their current and latest versions. This is basically an alias for composer show -lo.
The color coding is as such:
- green (=): Dependency is in the latest version and is up to date.
- yellow (~): Dependency has a new version available that includes backwards compatibility breaks according to semver, so upgrade when you can but it may involve work.
- red (!): Dependency has a new version that is semver-compatible and you should upgrade it.
remove
copy to clipboardcomposer remove vendor/package vendor/package2The remove command removes packages from the composer.json file from the current directory.
After removing the requirements, the modified requirements will be uninstalled.
Some composer.json keys explanation
minimum-stability
This defines the default behavior for filtering packages by stability. This defaults to stable, so if you rely on a dev package, you should specify it in your file to avoid surprises.All versions of each package are checked for stability, and those that are less stable than the minimum-stability setting will be ignored when resolving your project dependencies. (Note that you can also specify stability requirements on a per-package basis using stability flags in the version constraints that you specify in a require block (see package links for more details).
- Available options (in order of stability) are dev, alpha, beta, RC, and stable
require
Lists packages required by this package. The package will not be installed unless those requirements can be met.require-dev
Lists packages required for developing this package, or running tests, etc. The dev requirements of the root package are installed by default.Both install or update support the --no-dev option that prevents dev dependencies from being installed.
config
the list of parameters for config key can be found there : composer.json configfor example the key optimize-autoloader enable autoloader caching file for production environement.
Options
full cli command : composer cliCommand | Description |
---|---|
--prefer-source | There are two ways of downloading a package: source and dist. For stable versions Composer will use the dist by default. The source is a version control repository. If --prefer-source is enabled, Composer will install from source if there is one. This is useful if you want to make a bugfix to a project and get a local git clone of the dependency directly. |
--prefer-dist | Reverse of --prefer-source, Composer will install from dist if possible. This can speed up installs substantially on build servers and other use cases where you typically do not run updates of the vendors. It is also a way to circumvent problems with git if you do not have a proper setup. |
--dry-run | If you want to run through an installation without actually installing a package, you can use --dry-run. This will simulate the installation and show you what would happen. |
--dev | Install packages listed in require-dev (this is the default behavior). |
--no-dev | Skip installing packages listed in require-dev. The autoloader generation skips the autoload-dev rules. |
--no-autoloader | Skips autoloader generation. |
--no-scripts | Skips execution of scripts defined in composer.json. |
--no-progress | Removes the progress display that can mess with some terminals or scripts which don't handle backspace characters. |
--no-suggest | Skips suggested packages in the output. |
--optimize-autoloader (-o) | Convert PSR-0/4 autoloading to classmap to get a faster autoloader. This is recommended especially for production, but can take a bit of time to run so it is currently not done by default. |
--classmap-authoritative (-a) | Autoload classes from the classmap only. Implicitly enables --optimize-autoloader. |
--apcu-autoloader | Use APCu to cache found/not-found classes. |
--ignore-platform-reqs | ignore php, hhvm, lib-* and ext-* requirements and force the installation even if the local machine does not fulfill these. See also the platform config option. |
Example
copy to clipboard{ "name" : "frogg/devtool", "version" : "v1.0.0", "description" : "Common third-party tool storing folder", "type" : "library", "license" : "none", "authors": [ { "name" : "Frogg", "email": "admin@frogg.fr" } ], "support": { "email": "support@frogg.fr", "form" : "https://tool.frogg.fr/Contact" } "minimum-stability": "stable", "require": { "php" : "^7.2" } "require-dev": { "phpmd/phpmd" : "@stable", "sebastian/phpcpd" : "@stable", "phpdocumentor/phpdocumentor" : "@stable", "squizlabs/php_codesniffer" : "@stable" } "autoload": { "psr-4": { "App\\" : "inc/" } } }Full schema : composer.json schema