Symfony Routing
Beautiful URLs are a must for any serious web application. This means leaving behind ugly URLs like index.php?article_id=57 in favor of something like /read/intro-to-symfony.
Having flexibility is even more important. What if you need to change the URL of a page from /blog to /news? How many links would you need to hunt down and update to make the change? If you're using Symfony's router, the change is simple.
Official website : Symfony Routing
To test a first page it require to create this DefaultController class in src/controller/ for example.
copy to clipboard
copy to clipboard
Visualization of all routes (dev & prod) :
copy to clipboard
Visualization of a specific route :
copy to clipboard
Having flexibility is even more important. What if you need to change the URL of a page from /blog to /news? How many links would you need to hunt down and update to make the change? If you're using Symfony's router, the change is simple.
Official website : Symfony Routing
routes.yaml
this is the default configuration uncommented : copy to clipboardindex: path: / controller: App\Controller\DefaultController::indexThis create a route named index which redirect the url / to the method index of the class DefaultController which namespace is App\Controller
To test a first page it require to create this DefaultController class in src/controller/ for example.
namespace App\Controller; class IndexController { public function index() { return new Response("<html><body>FIRST SYMFONY PAGE !</body></html>"); } }
- Note that the method will return an object of type Response which include header & page content.
Using the import of Symfony\Component\HttpFoundation\Response;
-
This will redirect only the url /, the call of /index.php will not work !
It require to redirect /index.php to / in the server configuration.
Routes redirection
Dynamic redirection
copy to clipboard#route redirection home: path: /home controller: Symfony\Bundle\FrameworkBundle\Controller\RedirectController::redirectAction defaults: route: index permanent: trueWill redirect permanently(302) the url /home to the route named index which is / in the routes.yaml.
Static redirection
copy to clipboard#static redirection static_page: path: /static-page controller: Symfony\Bundle\FrameworkBundle\Controller\TemplateController::templateAction defaults: template: static/static_page.html.twigWill create a route named static_page which redirect the url /static-page to the template named static/static_page.html.twig.
Annotations (basis)
The routes can be directly added into the annotation (doc bloc) and will be understoud by the application using the command @Route.copy to clipboard
/** * @Route("/", name="index") * @return Response */ public function index() : Response { return new Response("<html><body>FIRST SYMFONY PAGE !</body></html>"); }In this example, the request / will call the method index.
- Route annotation require the import : use Symfony\Component\Routing\Annotation\Route;
Annotations (advanced)
copy to clipboard/** * @Route("/category/{label}_{id}", name="index_category", methods={"GET"},requirements={"id" : "\d+"}, defaults={"id" : "1"}) * @param $id * @return Response */ public function category(string $label, string $id) { return new Response(""<html><body>CATEGORY = $id !</body></html>""); }create a route with :
/category/{label}_{id} | route url structure with 2 variables $label and $id |
name="index_category" | name of the route |
methods={"GET"} | method of the lient request |
requirements={"id" : "\d+"} | condition to make the route valide, here $id must be at least one decimal (regexp) |
defaults={"id" : "1"} | default value for $id, if not $id is specified |
- It is advisable to add a default value to prevent unwanted behaviors.
- If route condition does not match a 404 error page will occur.
Routes visualization (@@@TODO UPDATE SCREENSHOTS@@@)
Visualization of production routes :copy to clipboard
php bin/console debug:router --env=prod
Visualization of all routes (dev & prod) :
copy to clipboard
php bin/console debug:router
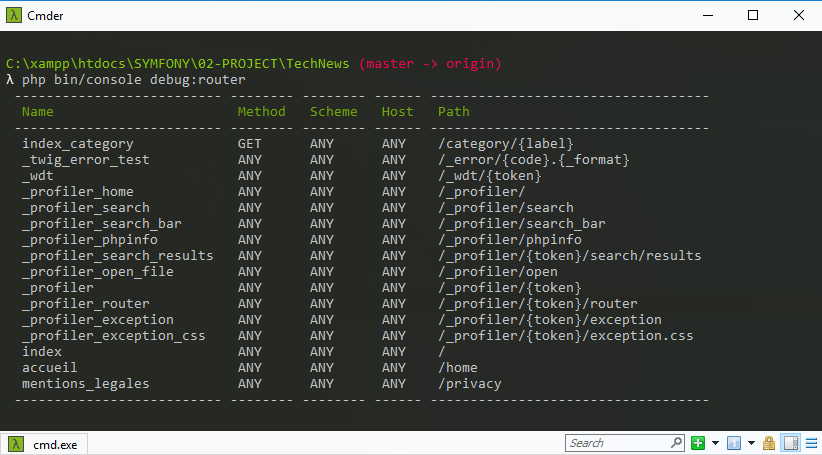
Visualization of a specific route :
copy to clipboard
php bin/console router:match /category/test
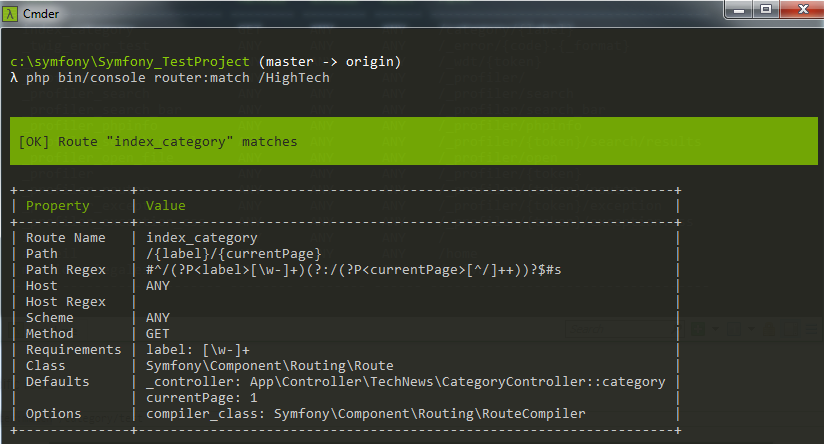