Symfony Console
The Symfony framework provides lots of commands through the bin/console script (e.g. the well-known bin/console cache:clear command). These commands are created with the Console component. You can also use it to create your own commands.
The list of available commands can be display using the command:
Official website : Symfony Console
The list of available commands can be display using the command:
php bin/console
Official website : Symfony Console
Usage
command [options] [arguments]
Options
-h, --help | Display this help message |
-q, --quiet | Do not output any message |
-V, --version | Display this application version |
--ansi | Force ANSI output |
--no-ansi | Disable ANSI output |
-n, --no-interaction | Do not ask any interactive question |
-e, --env=ENV | The Environment name. [default: "dev"] |
--no-debug | Switches off debug mode. |
-v|vv|vvv, --verbose | Increase the verbosity of messages: 1 for normal output, 2 for more verbose output and 3 for debug |
Commands
about | Displays information about the current project |
help | Displays help for a command |
list | Lists commands |
assets | |
---|---|
assets:install | Installs bundles web assets under a public directory |
cache | |
cache:clear | Clears the cache |
cache:pool:clear | Clears cache pools |
cache:pool:prune | Prune cache pools |
cache:warmup | Warms up an empty cache |
config | |
config:dump-reference | Dumps the default configuration for an extension |
debug | |
debug:autowiring | Lists classes/interfaces you can use for autowiring |
debug:config | Dumps the current configuration for an extension |
debug:container | Displays current services for an application |
debug:event-dispatcher | Displays configured listeners for an application |
debug:router | Displays current routes for an application |
debug:twig | Shows a list of twig functions, filters, globals and tests |
lint | |
lint:yaml | Lints a file and outputs encountered errors |
lint:twig | Lints a template and outputs encountered errors |
router | |
router:match | Helps debug routes by simulating a path info match |
doctrine | |
doctrine:cache:clear-collection-region | Clear a second-level cache collection region |
doctrine:cache:clear-entity-region | Clear a second-level cache entity region |
doctrine:cache:clear-metadata | Clears all metadata cache for an entity manager |
doctrine:cache:clear-query | Clears all query cache for an entity manager |
doctrine:cache:clear-query-region | Clear a second-level cache query region |
doctrine:cache:clear-result | Clears result cache for an entity manager |
doctrine:cache:contains | Check if a cache entry exists |
doctrine:cache:delete | Delete a cache entry |
doctrine:cache:flush | [doctrine:cache:clear] Flush a given cache |
doctrine:cache:stats | Get stats on a given cache provider |
doctrine:database:create | Creates the configured database |
doctrine:database:drop | Drops the configured database |
doctrine:database:import | Import SQL file(s) directly to Database. |
doctrine:ensure-production-settings | Verify that Doctrine is properly configured for a production environment |
doctrine:generate:entities | [generate:doctrine:entities] Generates entity classes and method stubs from your mapping information |
doctrine:mapping:convert | [orm:convert:mapping] Convert mapping information between supported formats |
doctrine:mapping:import | Imports mapping information from an existing database |
doctrine:mapping:info | |
doctrine:migrations:diff | Generate a migration by comparing your current database to your mapping information. |
doctrine:migrations:execute | Execute a single migration version up or down manually. |
doctrine:migrations:generate | Generate a blank migration class. |
doctrine:migrations:latest | Outputs the latest version number |
doctrine:migrations:migrate | Execute a migration to a specified version or the latest available version. |
doctrine:migrations:status | View the status of a set of migrations. |
doctrine:migrations:version | Manually add and delete migration versions from the version table. |
doctrine:query:dql | Executes arbitrary DQL directly from the command line |
doctrine:query:sql | Executes arbitrary SQL directly from the command line. |
doctrine:schema:create | Executes (or dumps) the SQL needed to generate the database schema |
doctrine:schema:drop | Executes (or dumps) the SQL needed to drop the current database schema |
doctrine:schema:update | Executes (or dumps) the SQL needed to update the database schema to match the current mapping metadata |
doctrine:schema:validate | Validate the mapping files |
make | |
make:auth | Creates an empty Guard authenticator |
make:command | Creates a new console command class |
make:controller | Creates a new controller class |
make:entity | Creates a new Doctrine entity class |
make:fixtures | Creates a new class to load Doctrine fixtures |
make:form | Creates a new form class |
make:functional-test | Creates a new functional test class |
make:migration | Creates a new migration based on database changes. |
make:serializer:encoder | Creates a new serializer encoder class |
make:subscriber | Creates a new event subscriber class |
make:twig-extension | Creates a new Twig extension class |
make:unit-test | Creates a new unit test class |
make:validator | Creates a new validator and constraint class |
make:voter | Creates a new security voter class |
server | |
server:log | Starts a log server that displays logs in real time |
server:run | Runs a local web server |
server:start | Starts a local web server in the background |
server:status | Outputs the status of the local web server for the given address |
server:stop | Stops the local web server that was started with the server:start command |
- The list of commands may vary in function of your components installed.
Example
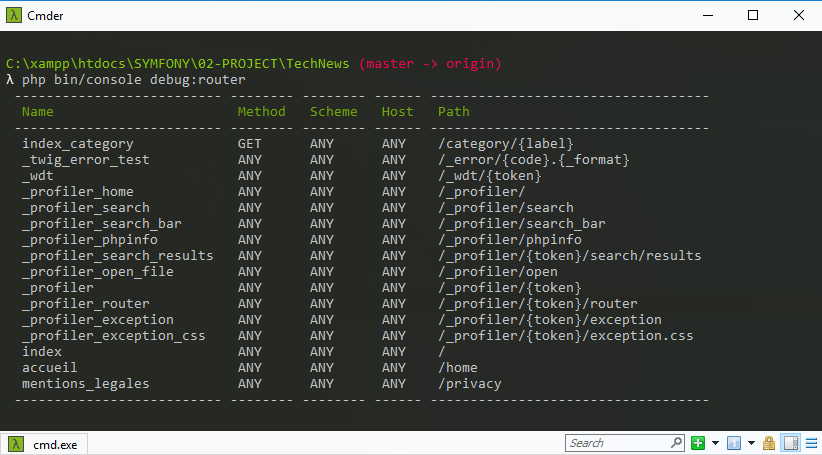
Creating a Command
Official website : Symfony Console
Commands are defined in classes extending Command. For example, you may want a command to create a user:
will display
Commands are defined in classes extending Command. For example, you may want a command to create a user:
Example of command
// src/Command/CreateUserCommand.php namespace App\Command; use Symfony\Component\Console\Command\Command; use Symfony\Component\Console\Input\InputInterface; use Symfony\Component\Console\Output\OutputInterface; class CreateUserCommand extends Command { protected function configure() { $this // the name of the command (the part after "bin/console") ->setName('app:create-user') // the short description shown while running "php bin/console list" ->setDescription('Creates a new user.') // the full command description shown when running the command with // the "--help" option ->setHelp('This command allows you to create a user...') ; } protected function execute(InputInterface $input, OutputInterface $output) { // outputs multiple lines to the console (adding "\n" at the end of each line) $output->writeln([ 'User Creator', '============', '', ]); // outputs a message followed by a "\n" $output->writeln('Whoa!'); // outputs a message without adding a "\n" at the end of the line $output->write('You are about to '); $output->write('create a user.'); } }
Executing the command
php bin/console app:create-user
will display
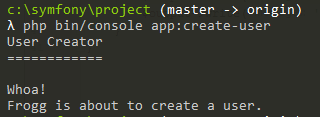
Console command updated
As you can see the new commands will apear in App section :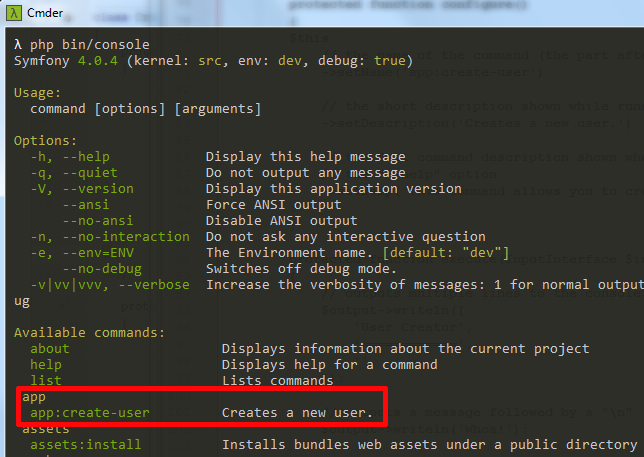
Clear all cache
It can occur that a php error page is due to the cache files.
Before loosing hour in trying to debug a working code, make sure you clear the cache : copy to clipboard
Before loosing hour in trying to debug a working code, make sure you clear the cache : copy to clipboard
composer dump-autoload php bin/console cache:clear