Symfony Basics
Symfony has published a new "skeleton" project, which is a minimal Symfony project recommended to create new applications.
This "skeleton" already includes Symfony Flex as a dependency. This means you can create a new Flex-enabled Symfony application by executing the following command:
copy to clipboard
Official website : Symfony Flex
An article is dedicted to phpSotrm, with a section for Symfony plugins : phpSotrm with Symfony
Most of the time, you'll be working in src/, templates/ or config/. As you keep reading, you'll learn what can be done inside each of these.
So what about the other directories in the project?
Official website : Symfony Setup
copy to clipboard
copy to clipboard
The virtual server can be displayed on the local address http://localhost:8000/
It also include some other usefull tool which will be seen later.
copy to clipboard
YAML (YAML Ain't Markup Language) is a human-readable data serialization language. It is commonly used for configuration files, but could be used in many applications where data is being stored (e.g. debugging output) or transmitted (e.g. document headers). YAML targets many of the same communications applications as XML but has a more minimal syntax which intentionally breaks compatibility with SGML. It uses both Python-style indentation to indicate nesting, and a more compact format that uses [] for lists and {} for maps making YAML 1.2 a superset of JSON.
Source : Wikipedia
Data structure hierarchy is maintained by outline indentation
example of default services.yaml :
To test your YAML files you can use an online validator like codebeautify.org/yaml-validator
This "skeleton" already includes Symfony Flex as a dependency. This means you can create a new Flex-enabled Symfony application by executing the following command:
copy to clipboard
composer create-project symfony/skeleton MyProjectFolderName
Official website : Symfony Flex
Adding Symfony plugin to phpStorm (optional but recommanded)
To work with Symfony there are plugins for phpStorm that help a lot for coding.An article is dedicted to phpSotrm, with a section for Symfony plugins : phpSotrm with Symfony
Folders Structure
Official website : Project Structure Global Structure presentation :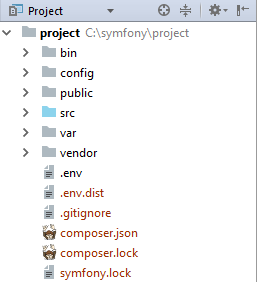
config/ | Contains... configuration of course!. You will configure routes, services and packages. |
src/ | All your PHP code lives here. |
templates/ | All your Twig templates live here. |
So what about the other directories in the project?
bin/ | The famous bin/console file lives here (and other, less important executable files). |
var/ | This is where automatically-created files are stored, like cache files (var/cache/) and logs (var/log/). |
vendor/ | Third-party (i.e. "vendor") libraries live here! These are downloaded via the Composer package manager. |
public/ | This is the document root for your project: you put any publicly accessible files here. And when you install new packages, new directories will be created automatically when needed. |
-
Symfony is using the Front Controller concept:
The front controller software design pattern is listed in several pattern catalogs and related to the design of web applications. It is "a controller that handles all requests for a website", which is a useful structure for web application developers to achieve the flexibility and reuse without code redundancy.
Which means, all request will be redirect on the default page index.php that will be the only accessible php file.
Other php files will be outside web root folder (public), so user can't try to display them by accessing their address in the url.
Running your Symfony Application (optional)
On production, you should use a web server like Nginx or Apache (see configuring a web server to run Symfony). But for development, it's even easier to use the Symfony PHP web server.Official website : Symfony Setup
copy to clipboard
composer require server --dev
copy to clipboard
php bin/console server:run
The virtual server can be displayed on the local address http://localhost:8000/
- Even if it can be a good solution for some people, I personnaly think this virtual server is too slow so I would prefere a real virtual server(With apache or Nginx) or even a XAMP
Symfony debug pack
This package will add a tool bar on the bottom of the web site which give usefull information for debug.It also include some other usefull tool which will be seen later.
copy to clipboard
composer require symfony/debugpack
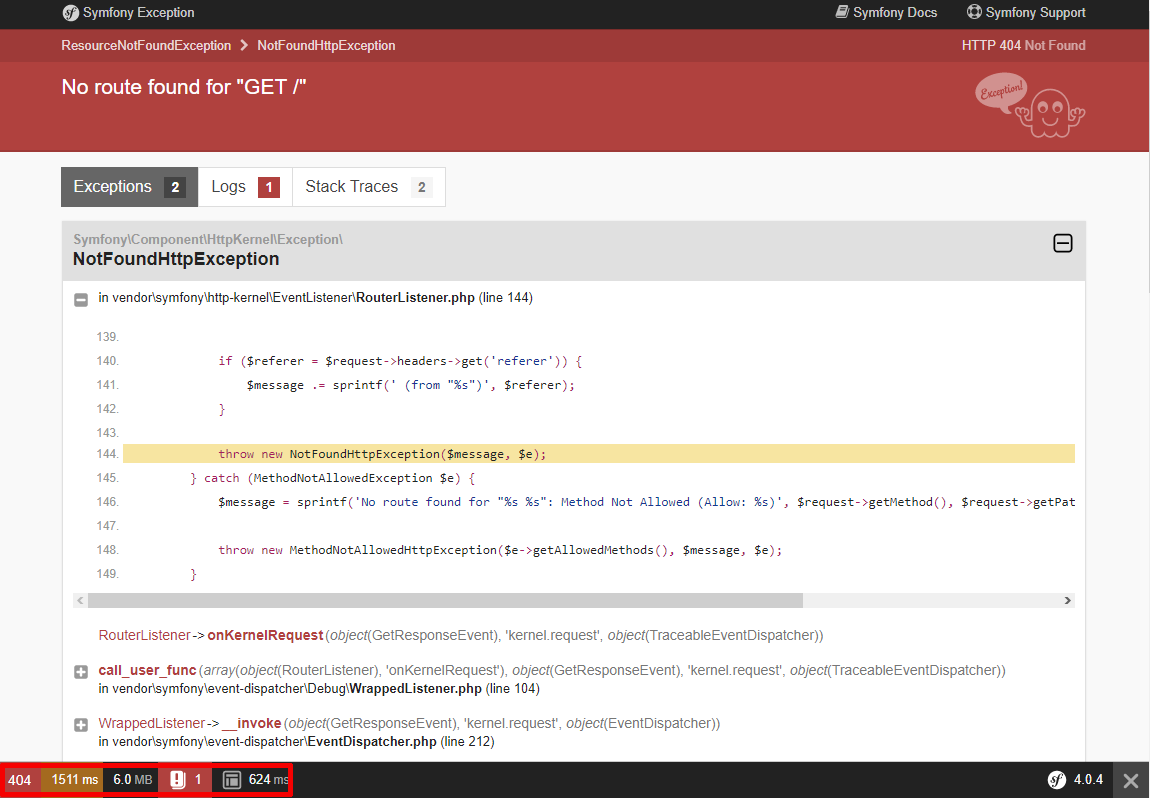
YAML files
Symfony use YAML files for its configuration files.YAML (YAML Ain't Markup Language) is a human-readable data serialization language. It is commonly used for configuration files, but could be used in many applications where data is being stored (e.g. debugging output) or transmitted (e.g. document headers). YAML targets many of the same communications applications as XML but has a more minimal syntax which intentionally breaks compatibility with SGML. It uses both Python-style indentation to indicate nesting, and a more compact format that uses [] for lists and {} for maps making YAML 1.2 a superset of JSON.
Source : Wikipedia
Data structure hierarchy is maintained by outline indentation
example of default services.yaml :
# Put parameters here that don't need to change on each machine where the app is deployed # https://symfony.com/doc/current/best_practices/configuration.html#application-related-configuration parameters: services: # default configuration for services in *this* file _defaults: autowire: true # Automatically injects dependencies in your services. autoconfigure: true # Automatically registers your services as commands, event subscribers, etc. public: false # Allows optimizing the container by removing unused services; this also means # fetching services directly from the container via $container->get() won't work. # The best practice is to be explicit about your dependencies anyway. # makes classes in src/ available to be used as services # this creates a service per class whose id is the fully-qualified class name App\: resource: '../src/*' exclude: '../src/{Entity,Migrations,Tests,Kernel.php}' # controllers are imported separately to make sure services can be injected # as action arguments even if you don't extend any base controller class App\Controller\: resource: '../src/Controller' tags: ['controller.service_arguments'] # add more service definitions when explicit configuration is needed # please note that last definitions always *replace* previous ones
- # in YAML files are used for comments
To test your YAML files you can use an online validator like codebeautify.org/yaml-validator