Third-party Tools (using composer)
Composer create a folder named vendor where all packages will be added
I will put all downloaded packages from composer in C:\dev\vendor
copy to clipboard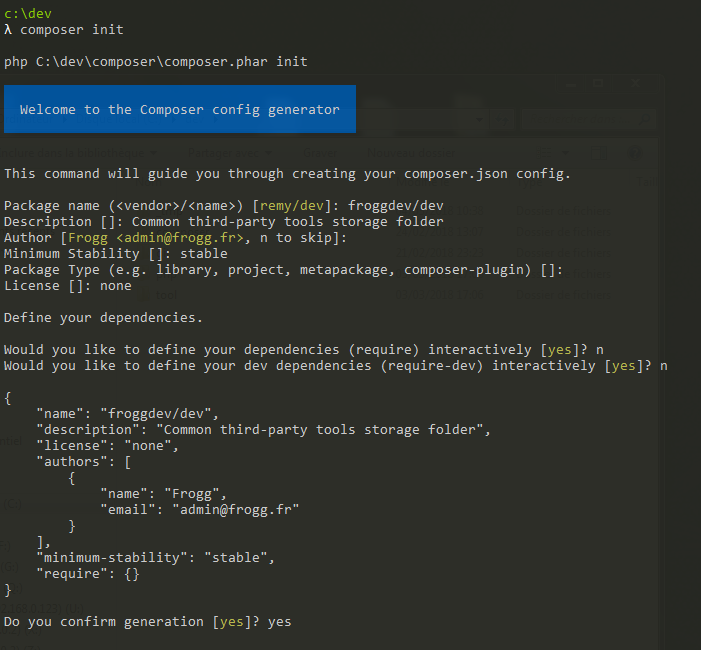
Once done a composer.json will be create and use for install third-party tool.
As this folder will be dedictated to phpStorm, it will not be linked directly with the others projects.
Wich mean packages install can be made without --dev option.
I will put all downloaded packages from composer in C:\dev\vendor
- Do not forget to make the previous tips using composer.bat as shortcut to be able to use the command "composer" without specifing php and the full composer path
- Linux version need some adaptation but it is almost the same things
Initializing composer folder (optional)
in a dos prompt go to C:\dev\ and init composer using the command :copy to clipboard
composer init
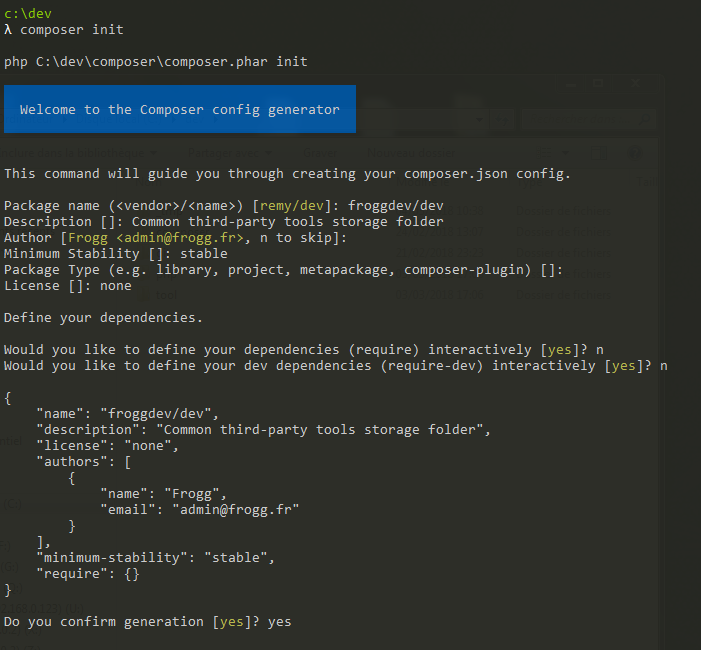
Once done a composer.json will be create and use for install third-party tool.
As this folder will be dedictated to phpStorm, it will not be linked directly with the others projects.
Wich mean packages install can be made without --dev option.
phpStorm external tool
This is how to add an external tool in phpStorm :
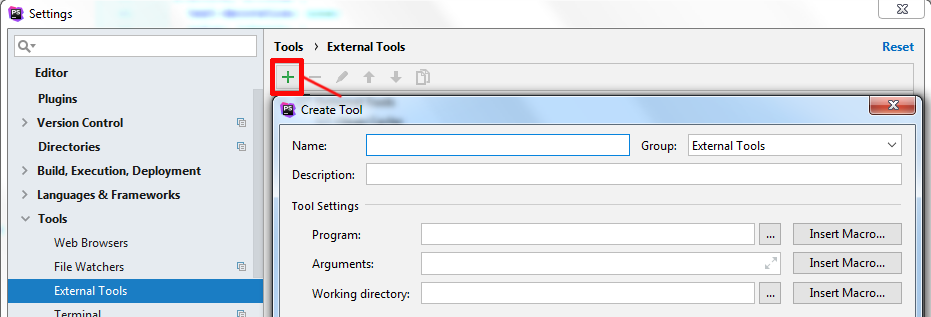
Php Metrics
PHP Metrics provides tons of metrics:
Official download phpmetrics.org
Html report command : copy to clipboard
To start inspection go to Tools > External Tools > PHP Metrics
Program : copy to clipboard
Arguments : copy to clipboard
- Complexity: Cyclomatic complexity, Myer's interval, Relative system complexity
- Volume: Vocabulary, Data complexity, Lines of code, Readability...
- Object Oriented: Lack of cohesion of methods, Coupling, Abstraction...
- Maintainability: Maintainability index, Halstead's metrics, Effort...
Official download phpmetrics.org
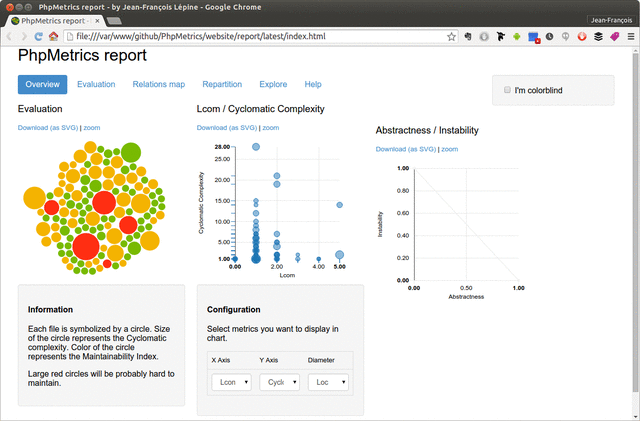
Php Metrics installation
To install it using composer, you can run the composer require command ( in c:\dev\ in my case ) : copy to clipboardcomposer require phpmetrics/phpmetrics
Html report command : copy to clipboard
php C:\dev\vendor\phpmetrics\phpmetrics\bin\phpmetrics --report-html={EXPORTFOLDER} {PHPSOURCESFOLDER}
PHP Metrics configuration
To add an external tool open : Settings > Tools > External tools
To start inspection go to Tools > External Tools > PHP Metrics
Program : copy to clipboard
php
Arguments : copy to clipboard
C:\dev\vendor\phpmetrics\phpmetrics\bin\phpmetrics --report-html=$ProjectFileDir$\public\output\metrics $ProjectFileDir$\src
- $ProjectFileDir$ mean the current project folder in phpStorm, like that, the config will be valid for any project without changing the configuration.
Php Mess Detector
It takes a given PHP source code base and look for several potential problems within that source. These problems can be things like:
Official download phpmd.org
Html report command : copy to clipboard
Then configure desired inspected files in the phpStorm tab code > Inspect Code
To start the full inspection go to code > Inspect Code tab, then click ok in the opened window

To start inspection go to Tools > External Tools > PHP Mess detector
Program : copy to clipboard
Arguments : copy to clipboard
- Possible bugs
- Suboptimal code
- Overcomplicated expressions
- Unused parameters, methods, properties
Official download phpmd.org
Php Mess Detector installation
To install it using composer, you can run the composer require command ( in c:\dev\ in my case ) : copy to clipboardcomposer require phpmd/phpmd
Html report command : copy to clipboard
php C:\dev\vendor\phpmd\phpmd\src\bin\phpmd {PHPSOURCESFOLDER} html cleancode,codesize,controversial,design,naming,unusedcode > {EXPORTEDFILEPATH}.html
- There is multiple export format available like text, xml, html...
PHP Mess Detector configuration
First you need to edit phpmd.bat in /bin from /vendor to set your php path and phpmd path (in my case C:\dev\vendor\phpmd\phpmd\src\bin\phpmd.bat) :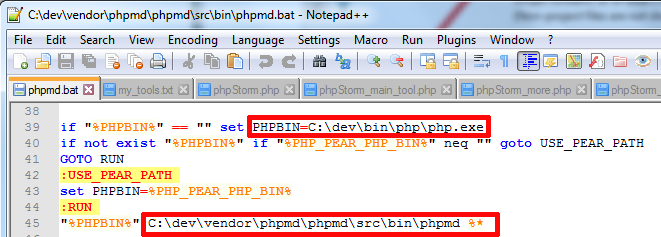
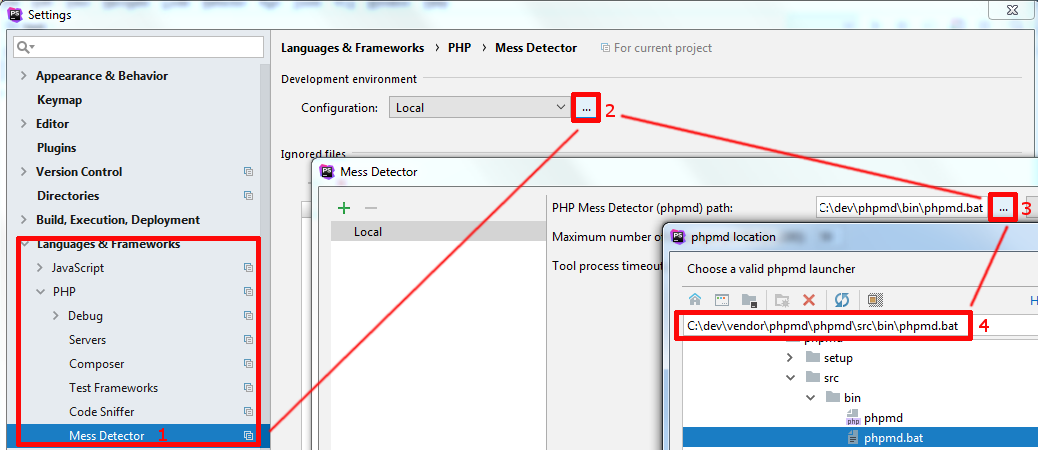
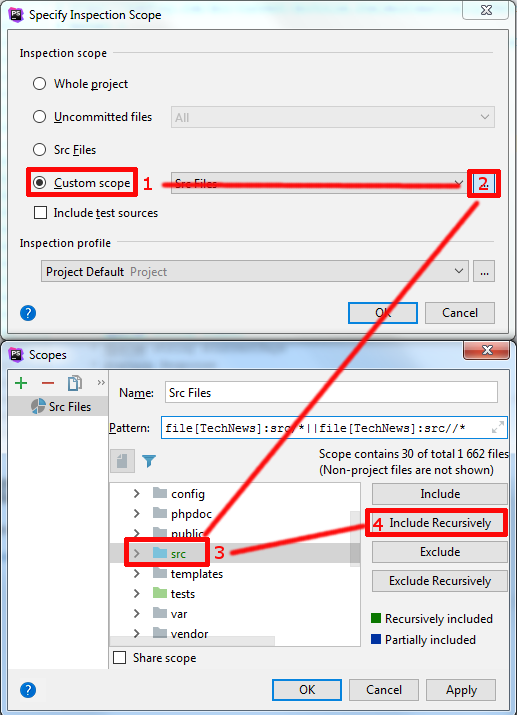
PHP Mess Detector configuration as external tool
To add an external tool open : Settings > Tools > External tools
To start inspection go to Tools > External Tools > PHP Mess detector
Program : copy to clipboard
php
Arguments : copy to clipboard
C:\dev\vendor\phpmd\phpmd\src\bin\phpmd $ProjectFileDir$\src html cleancode,codesize,controversial,design,naming,unusedcode > $ProjectFileDir$\public\output\phpmd.html
Php Code Sniffer & Code Beautifier and Fixer
PHP_CodeSniffer is a set of two PHP scripts; the main phpcs script that tokenizes PHP, JavaScript and CSS files to detect violations of a defined coding standard, and a second phpcbf script to automatically correct coding standard violations. PHP_CodeSniffer is an essential development tool that ensures your code remains clean and consistent.
Official download www.squizlabs.com/php-codesniffer
Txt report command : copy to clipboard
Automatically fix Coding standard command :
copy to clipboard

To start inspection go to Tools > External Tools > PHP Code Sniffer
Program : copy to clipboard
Arguments : copy to clipboard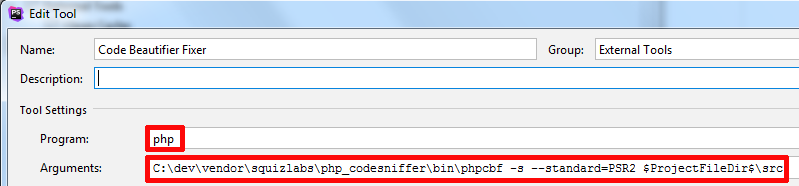
To start inspection go to Tools > External Tools > PHP Code Beautifier and Fixer
Program : copy to clipboard
Arguments : copy to clipboard
Official download www.squizlabs.com/php-codesniffer
Php Code Sniffer installation
To install it using composer, you can run the composer require command ( in c:\dev\ in my case ) : copy to clipboardcomposer require squizlabs/php_codesniffer
Txt report command : copy to clipboard
php C:\dev\vendor\squizlabs\php_codesniffer\bin\phpcs -s --standard=PSR2 --report=summary {PHPSOURCESFOLDER} > {EXPORTEDFILEPATH}.txt
-
Php Code Sniffer require xdebug, it looks into php.ini configuration to check the xdebug path adding php_{zend_extension}.dll so you need to configure the zend extension without the php_ or .dll at the end to prevent trouble of localization:
correct : zend_extension='xdebug-2.6.0-7.2-vc15-x86_64'
error : zend_extension='php_xdebug-2.6.0-7.2-vc15-x86_64'
copy to clipboard
php C:\dev\vendor\squizlabs\php_codesniffer\bin\phpcbf -s --standard=PSR2 {PHPSOURCESFOLDER}
PHP Code Sniffer configuration
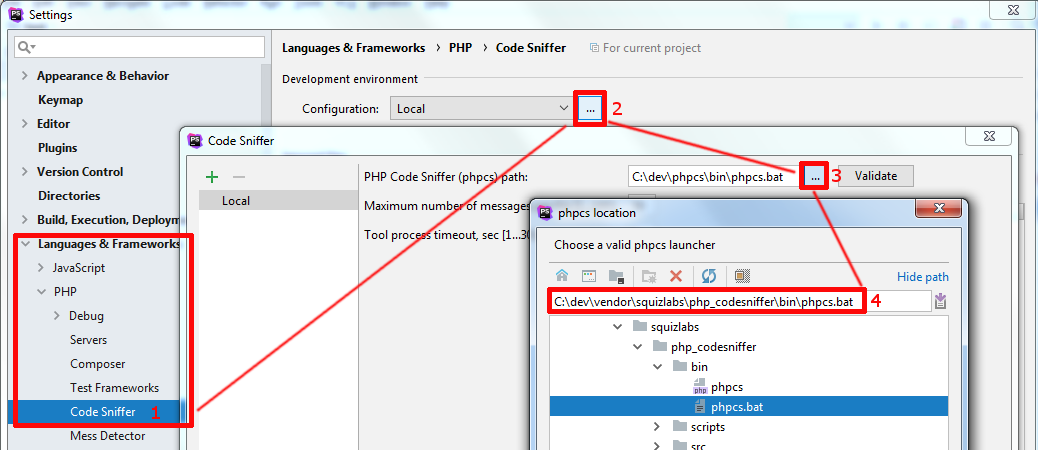
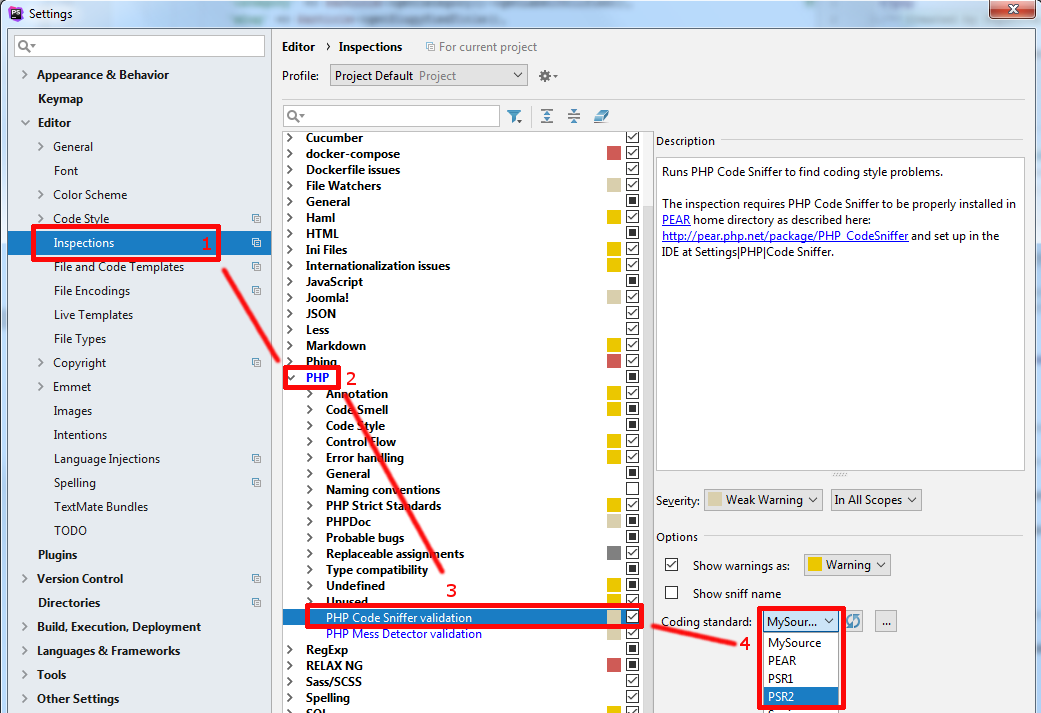
PHP Code Sniffer configuration as external tool
To add an external tool open : Settings > Tools > External tools
To start inspection go to Tools > External Tools > PHP Code Sniffer
Program : copy to clipboard
php
Arguments : copy to clipboard
C:\dev\vendor\squizlabs\php_codesniffer\bin\phpcs -s --standard=PSR2 --report=summary $ProjectFileDir$\src > $ProjectFileDir$\public\output\phpcs.txt
PHP Code Beautifier and Fixer configuration as external tool
To add an external tool open : Settings > Tools > External tools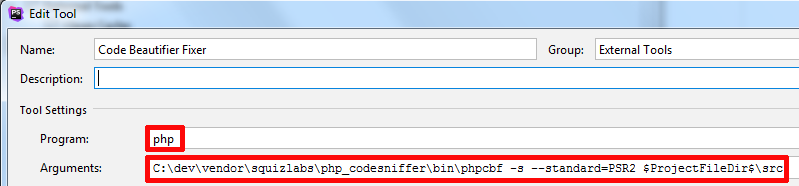
To start inspection go to Tools > External Tools > PHP Code Beautifier and Fixer
Program : copy to clipboard
php
Arguments : copy to clipboard
C:\dev\vendor\squizlabs\php_codesniffer\bin\phpcbf -s --standard=PSR2 $ProjectFileDir$\src
PHP Copy/Paste Detector
phpcpd is a Copy/Paste Detector (CPD) for PHP code.
Used to factorize the code and prevent duplicated code.
Official download github.com/sebastianbergmann/phpcpd/
Txt report command : copy to clipboard
To start inspection go to tool > external tool > PHP Copy/Paste Detector
Program : copy to clipboard
Arguments : copy to clipboard
Used to factorize the code and prevent duplicated code.
Official download github.com/sebastianbergmann/phpcpd/
PHP Copy/Paste Detector installation
To install it using composer, you can run the composer require command ( in c:\dev\ in my case ) : copy to clipboardcomposer require sebastian/phpcpd
Txt report command : copy to clipboard
php C:\dev\vendor\sebastian\phpcpd\phpcpd $ProjectFileDir$\src
PHP Copy/Paste Detector configuration
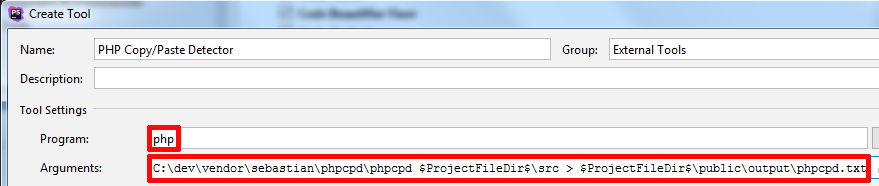
Program : copy to clipboard
php
Arguments : copy to clipboard
C:\dev\vendor\sebastian\phpcpd\phpcpd $ProjectFileDir$\src > $ProjectFileDir$\public\output\phpcpd.txt
PHP Depend
PHP_Depend is a small program that performs static code analysis on a given source base. Static code analysis means that PHP_Depend first takes the source code and parses it into an easily processable internal data structure. This data structure is normally called an AST (Abstract Syntax Tree), that represents the different statements and elements used in the analyzed source base. Then it takes the generated AST and measures several values, the so called software metrics. Each of this values stands for a quality aspect in the the analyzed software, observed from a very high level of abstraction, because no source was reviewed manually until now.
Official download pdepend.org
Report command : copy to clipboard
Program : copy to clipboard
Arguments :
copy to clipboard
Official download pdepend.org
PHP Depend installation
To install it using composer, you can run the composer require command ( in c:\dev\ in my case ) : copy to clipboardcomposer require pdepend/pdepend
Report command : copy to clipboard
php C:\dev\vendor\pdepend\pdepend\src\bin\pdepend --summary-xml={EXPORTEDFILEPATH}}.xml --jdepend-chart={EXPORTEDFILEPATH}}.svg --overview-pyramid={EXPORTEDFILEPATH}}.svg {PHPSOURCESFOLDER}
PHP Depend configuration
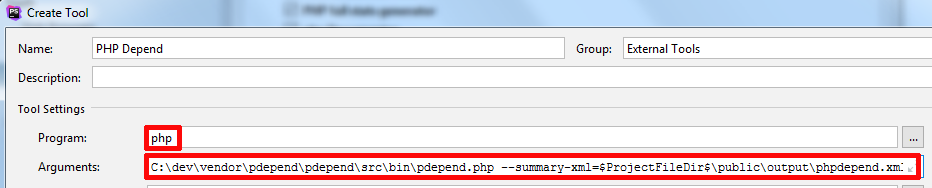
Program : copy to clipboard
php
Arguments :
copy to clipboard
C:\dev\vendor\pdepend\pdepend\src\bin\pdepend.php --summary-xml=$ProjectFileDir$\public\output\phpdepend.xml --jdepend-chart=$ProjectFileDir$\public\output\jdepend.svg --overview-pyramid=$ProjectFileDir$\public\output\pyramid.svg $ProjectFileDir$\src
PHP Documentor
phpDocumentor is a tool that makes it possible to generate documentation directly from your PHP source code. With this you can provide your consumers with more information regarding the functionality embedded within your source and not just what is usable to them from your user interface.
Official download www.phpdoc.org
Report command : copy to clipboard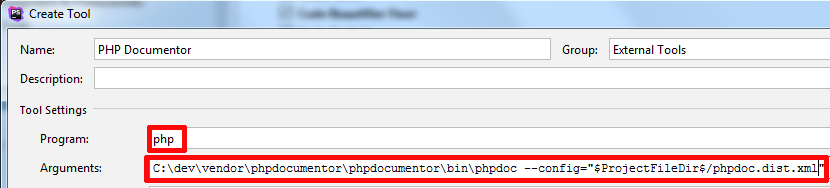
File Template for phpdoc.dist.xml
copy to clipboard
Official download www.phpdoc.org
PHP Documentor installation
To install it using composer, you can run the composer require command ( in c:\dev\ in my case ) : copy to clipboardcomposer require phpdocumentor/phpdocumentor
Report command : copy to clipboard
C:\dev\vendor\phpdocumentor\phpdocumentor\bin\phpdoc -t $ProjectFileDir$\public\output\phpdoc -d $ProjectFileDir$\src\
PHP Documentor configuration
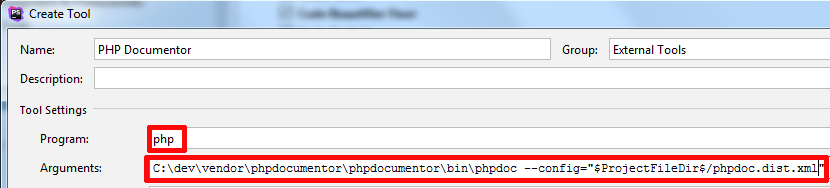
File Template for phpdoc.dist.xml
copy to clipboard
<?xml version="1.0" encoding="UTF-8" ?> <phpdoc> <title>${PROJECT_NAME}</title> <parser> <target>public/output/phpdoc</target> <encoding>utf8</encoding> <extensions> <extension>module</extension> <extension>inc</extension> <extension>php</extension> </extensions> </parser> <transformer> <target>public/output/phpdoc/</target> </transformer> <files> <directory>src</directory> </files> <logging> <level>warn</level> <paths> <default>public/output/phpdoc/log/{DATE}.log</default> <errors>public/output/phpdoc/log/{DATE}.errors.log</errors> </paths> </logging> </phpdoc>
Full stats generator script
the script is available here :
This script use all previous packages.
example:
Program : copy to clipboard
Arguments :
copy to clipboard
Script configuration
REM GLOBAL CONFIG VARS REM ------------------------- REM project path set vendor=C:\dev\vendor set index=index.html REM -------------------------
- vendor : path to vendor folder
- index : file name result
Script command
script command :export.bat ProjectPath sourceFolder webFolder outputFolder
example:
export.bat $ProjectFileDir$ src public outputthis will create stats in public/output/index.html
Script configuration as external tool
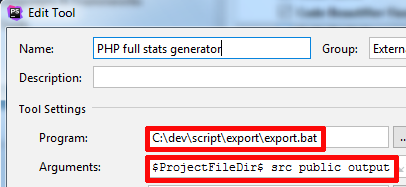
Program : copy to clipboard
C:\dev\script\export\export.bat
Arguments :
copy to clipboard
$ProjectFileDir$ src public output